2022. 12. 30. 20:35ㆍJava/coding test
Fish
N voracious fish are moving along a river. Calculate how many fish are alive.
You are given two non-empty arrays A and B consisting of N integers. Arrays A and B represent N voracious fish in a river, ordered downstream along the flow of the river.
The fish are numbered from 0 to N − 1. If P and Q are two fish and P < Q, then fish P is initially upstream of fish Q. Initially, each fish has a unique position.
Fish number P is represented by A[P] and B[P]. Array A contains the sizes of the fish. All its elements are unique. Array B contains the directions of the fish. It contains only 0s and/or 1s, where:
- 0 represents a fish flowing upstream,
- 1 represents a fish flowing downstream.
If two fish move in opposite directions and there are no other (living) fish between them, they will eventually meet each other. Then only one fish can stay alive − the larger fish eats the smaller one. More precisely, we say that two fish P and Q meet each other when P < Q, B[P] = 1 and B[Q] = 0, and there are no living fish between them. After they meet:
- If A[P] > A[Q] then P eats Q, and P will still be flowing downstream,
- If A[Q] > A[P] then Q eats P, and Q will still be flowing upstream.
We assume that all the fish are flowing at the same speed. That is, fish moving in the same direction never meet. The goal is to calculate the number of fish that will stay alive.
For example, consider arrays A and B such that:
A[0] = 4 B[0] = 0 A[1] = 3 B[1] = 1 A[2] = 2 B[2] = 0 A[3] = 1 B[3] = 0 A[4] = 5 B[4] = 0
Initially all the fish are alive and all except fish number 1 are moving upstream. Fish number 1 meets fish number 2 and eats it, then it meets fish number 3 and eats it too. Finally, it meets fish number 4 and is eaten by it. The remaining two fish, number 0 and 4, never meet and therefore stay alive.
Write a function:
class Solution { public int solution(int[] A, int[] B); }
that, given two non-empty arrays A and B consisting of N integers, returns the number of fish that will stay alive.
For example, given the arrays shown above, the function should return 2, as explained above.
Write an efficient algorithm for the following assumptions:
- N is an integer within the range [1..100,000];
- each element of array A is an integer within the range [0..1,000,000,000];
- each element of array B is an integer that can have one of the following values: 0, 1;
- the elements of A are all distinct.
풀이
A배열에는 물고기의 크기가, B배열에는 물고기가 움직이는 방향이 들어있습니다.
A배열의 인덱스가 작은 순으로 하천의 위에 위치합니다.
B배열에서 0은 아래에서 위로 움직이는 것이고 1은 위에서 아래로 움직이는 것입니다.
큰물고기는 작은 물고기를 잡아먹으며, 움직이는 방향은 변하지 않습니다.
최종적으로 살아있는 물고기의 수를 구하면 됩니다.
그림으로 설명해보자면 아래와 같은 단계를 거친다고 생각하면됩니다.
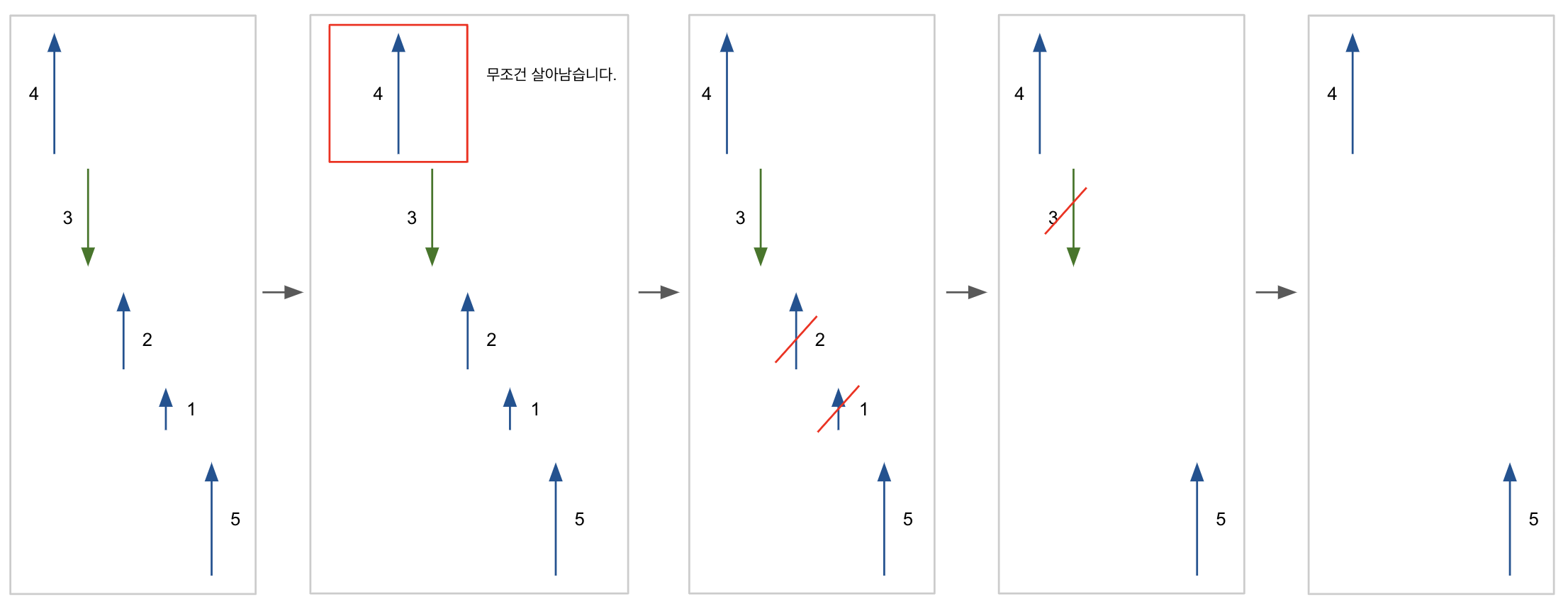
A: new int[]{4, 3, 2, 1, 5}
B: new int[]{0, 1, 0, 0, 0}
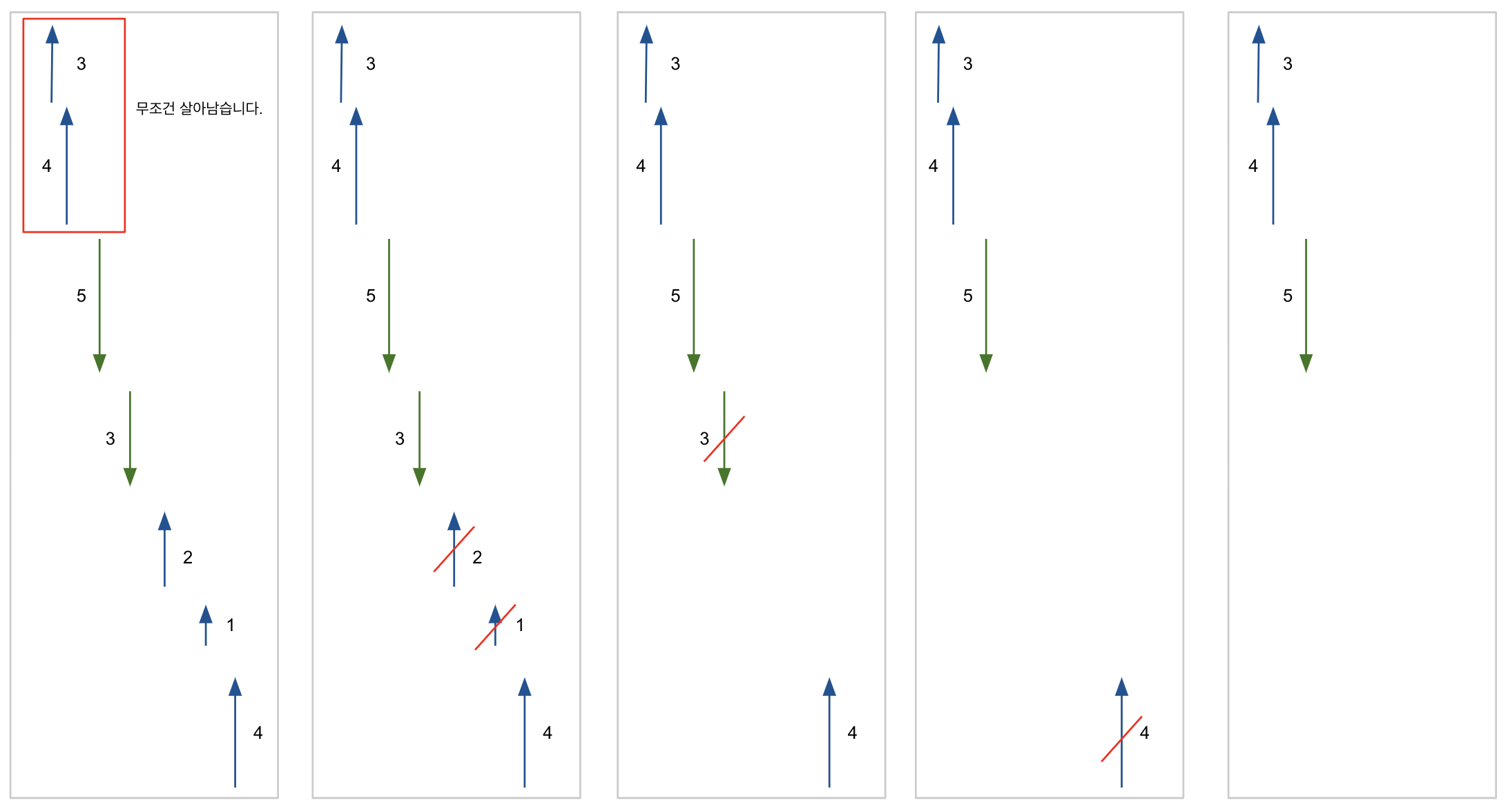
A: new int[]{3, 4, 5, 3, 2, 1, 4}
B: new int[]{0, 0, 1, 1, 0, 0, 0}
코드
static int fish(int[] A, int[] B) { int result = 0; Stack<Integer> stack = new Stack<>(); for (int i = 0; i < A.length; i++) { if (B[i] == 1) { stack.push(A[i]); } else { while (!stack.empty()) { if(stack.peek() < A[i]) { stack.pop(); } else { break; } } if (stack.empty()) { result++; } } } return result + stack.size(); }
'Java > coding test' 카테고리의 다른 글
[Codility - Java] 4. Counting Elements - 3. MaxCounters (0) | 2023.01.09 |
---|---|
[Codility - Java] 5. Prefix Sums - 2. CountDiv (0) | 2023.01.04 |
[Codility - Java] 5. Prefix Sums - 1. PassingCars (0) | 2023.01.04 |
[Codility - Java] 7. Stacks and Queues - 1. Brackets (0) | 2023.01.02 |
[Codility - Java] 7. Stacks and Queues - 3. Nesting (0) | 2023.01.02 |